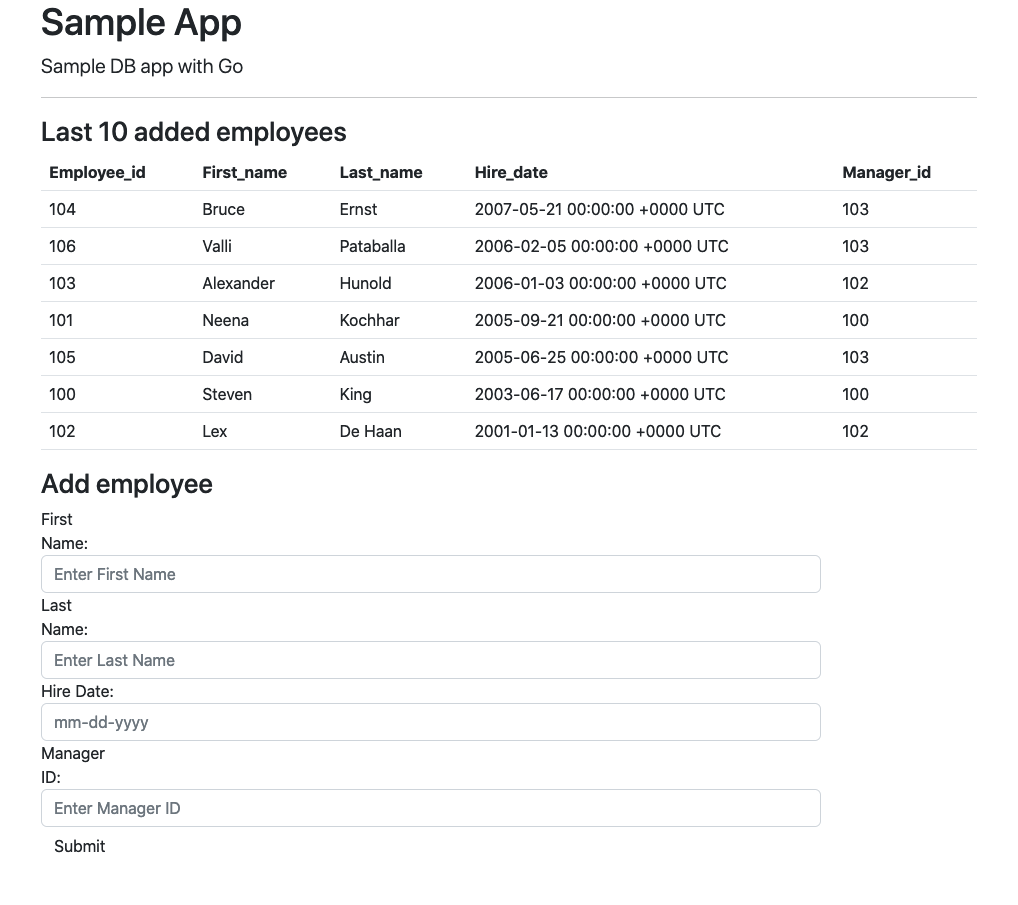
Yes, this is one more sample app as there are probably thousands already on the internet. We have tons of sample apps from different vendors with various types of licenses available on different repositories. Nevertheless sometimes I struggle to find exactly what I need – a simple app with a database backend which can work with Oracle Autonomous databases and optionally with Postgres backend. In my everyday life I primarily use Go as a programming language and I would like to have such an app written using that language. So, eventually I gave up and created my own application with a simple frontend and two (as for now) options for backend databases – Oracle and Postgres.
The application is available on Github and has a simple structure.
sample-app$ tree . ├── Dockerfile ├── LICENSE ├── README.md ├── cmd │ └── app │ └── main.go ├── connect_ora.go ├── connect_pg.go ├── data ├── dboper_ora.go ├── dboper_pg.go ├── go.mod ├── go.sum ├── ora_conn.log ├── sampleapp.go ├── sql │ └── hr_cre.sql └── ssl └── wallet.zip
The ./cmd/app/main.go is the main package which is calling the sampleapp package from the root directory to run the code. The sampleapp package is split to 5 files where the sampleapp.go is the main file and the four others represent functions for Postgres and Oracle backends. The application is using the go-ora driver for the Oracle database access and the pgx driver for Postgres.
The main index.html page is embedded to the sampleapp.go but that can be easily replaced by a more complex structure with directory and several files by changing a couple of variables and switching from “Parse” to “ParseFiles” functions in the code.
The sql directory has only one file hr_cre.sql to initialize the schema by creating a simple employees table and populating it by several records. As I said it is only a basic application which can be extended by different tables or building a much bigger dataset. One option is to use the Oracle sample schemas for that.
The ssl directory can be used for either certificates and keys for Postgres database (still in my todo list) or for a wallet file for Oracle SSL connection. It is not hardcoded and can be defined using environment variables.
As of now for simplicity the application is using a set of environment variables to define parameters and credentials for database connection but it can be replaced by any other proper solutions in a more mature environment. Here is the list of variables used by the application:
- DBVERSION – POSTGRESQL or ORACLE (POSTGRESQL by default)
- DBNAME – either Oracle service or Postgres database name
- DBHOST – either fully qualified host name or IP address for the database server
- DBPORT – listener port on DB side (5432 by default – Postgres, 1521 – Oracle)
- DBUSER – username for connection
- DBPASS – user password
- DBWALLET – path to the uncompressed Oracle wallet
Application can accept one parameter “-port” which defines the port where your http server listener will run. By default it runs on the port 8080.
How can you use the application? You have several options. Let’s go through some of them.
The first way is to run the application on your local machine or a VM where you have the Go environment installed. It is easy and requires only a few steps. Here is list of steps for an Oracle Linux VM:
$ sudo dnf update -y $sudo dnf install golang git -y $ git clone https://github.com/gotochkin/sample-app.git $ cd sample-app/ $ mkdir ssl ### Unzip your wallet file to the ssl directory $ unzip ~/Wallet_myatpdb.zip -d ssl/ #create a file with environment variables or export them explicitly $ vi ~/sampleapp.env $ cat ~/sampleapp.env ##################################################### DBNAME=m5c5hcat3eqqydh_myatpdb_tp.adb.oraclecloud.com DBWALLET=~/sample-app/ssl DBHOST=adb.us-ashburn-1.oraclecloud.com DBPORT=1522 DBPASS=MyExtremelyDifficultToRememberPassword DBUSER=sampleapp DBVERSION=ORACLE export DBNAME DBHOST DBPORT DBPASS DBUSER DBWALLET DBVERSION ##################################################### $ source ~/sampleapp.env #Enable port 8080 in firewall $ sudo firewall-cmd --permanent --zone=public --add-port=8080/tcp $ sudo firewall-cmd --reload #Now you can run it interactively executing $ go run cmd/app/main.go ... 2022/09/23 15:06:47 Listening on port :8080 #Or build it and run $ go build -v -o sampleapp ./cmd/app/ $ ./sampleapp 2022/09/23 15:31:31 Listening on port :8080
After that you can access it by connecting to http://localhost:8080

The second way is to use the included Dockerfile and build a container using local docker. If you have a look inside the docker file you can see that for Oracle SSL it is using the./ssl directory and the wallet file name wallet.zip. If you decide to use any other directory for the files you can modify it providing either relative or full path.
#Unzip wallet file - for oracle SSL. #Comment it if you don't have oracle ssl connection RUN apt-get update && apt-get install -y unzip && \ unzip ssl/wallet.zip -d ./ssl ... #Copy wallet files COPY --from=builder /sampleapp/ssl /sampleapp/ssl
You need to build the image and after building you need to pass the environment variables using either file with the variables or directly in the command line. In the example I’ve used a file where I placed all the variables.
$docker build -t otochkin/sampleapp:0.01 . docker run --name sampleapp -p 8080:8080 --env-file ssl/pg_env otochkin/sampleapp:0.01
Those are only a couple of ways to run it. You can deploy it as an application to your Kubernetes cluster on premises or in a cloud. I will be adding some other components in the future and might write another post about the deployment options. The application is still in development and requires a lot of work. I have a long todo list and here are some of the first changes I am working on.
- Postgres SSL connection
- Schema and table initialization during the first boot
- Unit testing
- Readme
- Adding response time for DML to the index.html page
- Deployment Yaml file
- Cloud deployments for various public cloud vendors
Be free to use and test the application and write to me if you hit any issues. The best way to connect with me is my twitter @sky_vst. I hope it might help somebody with the first steps in Go or a database backend development or with general tests and infrastructure assessments.